Class SimpleGlyphDataImpression
A "Glyphs" data impression that uses hand-sculpted geometry to depict point data.
Inheritance
Namespace: IVLab.ABREngine
Assembly: IVLab.ABREngine.Runtime.dll
Syntax
[ABRPlateType("Glyphs")]
public class SimpleGlyphDataImpression : DataImpression, IHasDataset, IHasKeyData, ICoordSpaceConverter
Examples
An example of creating a single glyph data impression and setting its colormap, color variable, and glyph could be:
SimpleGlyphDataImpression gi = new SimpleGlyphDataImpression();
gi.keyData = points;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.glyph = glyph;
ABREngine.Instance.RegisterDataImpression(gi);
Constructors
| Improve this Doc View SourceSimpleGlyphDataImpression()
A "Glyphs" data impression that uses hand-sculpted geometry to depict point data.
Declaration
protected SimpleGlyphDataImpression()
Examples
An example of creating a single glyph data impression and setting its colormap, color variable, and glyph could be:
SimpleGlyphDataImpression gi = new SimpleGlyphDataImpression();
gi.keyData = points;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.glyph = glyph;
ABREngine.Instance.RegisterDataImpression(gi);
Fields
| Improve this Doc View SourceLayerName
Define the layer name for this Data Impression
Declaration
protected const string LayerName = "ABR_Glyph"
Field Value
Type | Description |
---|---|
string |
Remarks
Warning
New Data Impressions should define a const string "LayerName" which corresponds to a Layer in Unity's Layer manager.
colorVariable
Scalar color variable applied to each point of this data impression. This example switches between X-axis monotonically increasing and Y-axis monotonically increasing.
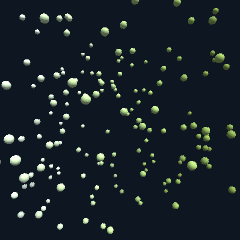
Declaration
[ABRInput("Color Variable", UpdateLevel.Style)]
public ScalarDataVariable colorVariable
Field Value
Type | Description |
---|---|
ScalarDataVariable |
colormap
Colormap applied to the colorVariable. This example switches between a linear white-to-green colormap and a linear black-to-white colormap.
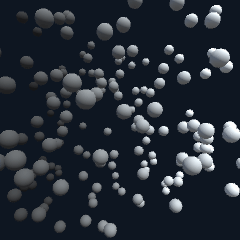
Declaration
[ABRInput("Colormap", UpdateLevel.Style)]
public IColormapVisAsset colormap
Field Value
Type | Description |
---|---|
IColormapVisAsset |
forceOutlineColor
Force the use of outlineColor even if there's a colormap applied to the data. This example alternates between a white-to-green linear colormap (false) and a solid purple-blue (true)
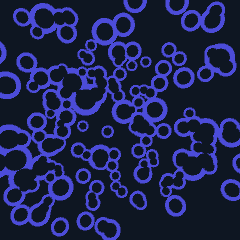
Declaration
[ABRInput("Force Outline Color", UpdateLevel.Geometry)]
public BooleanPrimitive forceOutlineColor
Field Value
Type | Description |
---|---|
BooleanPrimitive |
forwardVariable
"Forward" direction that glyphs should point in.
Declaration
[ABRInput("Forward Variable", UpdateLevel.Geometry)]
public VectorDataVariable forwardVariable
Field Value
Type | Description |
---|---|
VectorDataVariable |
glyph
What glyph(s) to apply to the dataset. This can also take a GlyphGradient. This example alternates between spherical and thin cylindrical glyphs.
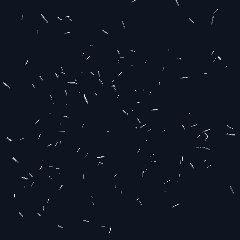
Declaration
[ABRInput("Glyph", UpdateLevel.Geometry)]
public IGlyphVisAsset glyph
Field Value
Type | Description |
---|---|
IGlyphVisAsset |
glyphDensity
Tweak the density of glyphs - subsamples the existing glyphs uniformly.
Declaration
[ABRInput("Glyph Density", UpdateLevel.Style)]
public PercentPrimitive glyphDensity
Field Value
Type | Description |
---|---|
PercentPrimitive |
glyphLod
Level of detail to use for glyph rendering (higher number = lower level of detail; most glyphs have 3 LODs). Default to using the second-highest level of detail, but you may need to adjust for performance reasons.
Declaration
[ABRInput("Glyph Level Of Detail", UpdateLevel.Geometry)]
public IntegerPrimitive glyphLod
Field Value
Type | Description |
---|---|
IntegerPrimitive |
glyphSize
Adjust the size of the glyphs (in Unity-space meters).
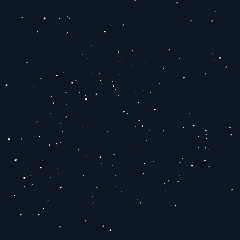
Declaration
[ABRInput("Glyph Size", UpdateLevel.Style)]
public LengthPrimitive glyphSize
Field Value
Type | Description |
---|---|
LengthPrimitive |
glyphVariable
Variable used to determine which glyph to render at which data values. This only has any effect if glyph is a GlyphGradient.
Declaration
[ABRInput("Glyph Variable", UpdateLevel.Style)]
public ScalarDataVariable glyphVariable
Field Value
Type | Description |
---|---|
ScalarDataVariable |
keyData
A "Glyphs" data impression that uses hand-sculpted geometry to depict point data.
Declaration
[ABRInput("Key Data", UpdateLevel.Geometry)]
public KeyData keyData
Field Value
Type | Description |
---|---|
KeyData |
Examples
An example of creating a single glyph data impression and setting its colormap, color variable, and glyph could be:
SimpleGlyphDataImpression gi = new SimpleGlyphDataImpression();
gi.keyData = points;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.glyph = glyph;
ABREngine.Instance.RegisterDataImpression(gi);
|
Improve this Doc
View Source
nanColor
Override the color used for NaN values in this data impression. If not supplied, will use the defaultNanColor.
Declaration
[ABRInput("NaN Color", UpdateLevel.Style)]
public IColormapVisAsset nanColor
Field Value
Type | Description |
---|---|
IColormapVisAsset |
outlineColor
Color of the outline
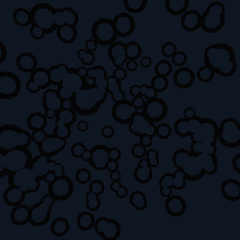
Declaration
[ABRInput("Outline Color", UpdateLevel.Geometry)]
public IColormapVisAsset outlineColor
Field Value
Type | Description |
---|---|
IColormapVisAsset |
outlineWidth
Width (in Unity world coords) of the outline
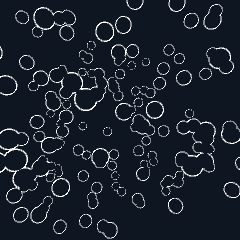
Declaration
[ABRInput("Outline Width", UpdateLevel.Geometry)]
public LengthPrimitive outlineWidth
Field Value
Type | Description |
---|---|
LengthPrimitive |
showOutline
Show/hide outline on this data impression
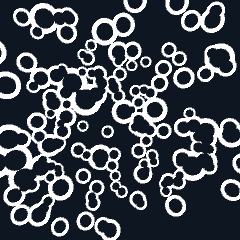
Declaration
[ABRInput("Show Outline", UpdateLevel.Geometry)]
public BooleanPrimitive showOutline
Field Value
Type | Description |
---|---|
BooleanPrimitive |
upVariable
"Up" direction that glyphs should point in.
Declaration
[ABRInput("Up Variable", UpdateLevel.Geometry)]
public VectorDataVariable upVariable
Field Value
Type | Description |
---|---|
VectorDataVariable |
useRandomOrientation
Use random forward/up directions when no Vector variables are applied for forward/up.
Declaration
[ABRInput("Use Random Orientation", UpdateLevel.Geometry)]
public BooleanPrimitive useRandomOrientation
Field Value
Type | Description |
---|---|
BooleanPrimitive |
Properties
| Improve this Doc View SourceMaterialNames
Name of the material to use to render this DataImpression
Declaration
protected override string[] MaterialNames { get; }
Property Value
Type | Description |
---|---|
string[] |
Overrides
Methods
| Improve this Doc View SourceCleanup()
When this data impression is done being used, clean up after itself if necessary. This method may need access to the GameObject the data impression is applied to.
Declaration
public override void Cleanup()
Overrides
| Improve this Doc View SourceComputeGeometry()
RENDERING STEP 1. Populate rendering information (Geometry) for the DataImpression. This is triggered by the DataImpressionGroup when an Geometry happens. This step is generally expensive.
Declaration
public override void ComputeGeometry()
Overrides
| Improve this Doc View SourceGetDataset()
By default, there's no dataset. DataImpressions should only have one dataset, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override Dataset GetDataset()
Returns
Type | Description |
---|---|
Dataset |
Overrides
| Improve this Doc View SourceGetKeyData()
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override KeyData GetKeyData()
Returns
Type | Description |
---|---|
KeyData |
Overrides
| Improve this Doc View SourceGetKeyDataTopology()
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override DataTopology GetKeyDataTopology()
Returns
Type | Description |
---|---|
DataTopology |
Overrides
| Improve this Doc View SourceSetKeyData(KeyData)
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override void SetKeyData(KeyData kd)
Parameters
Type | Name | Description |
---|---|---|
KeyData | kd |
Overrides
| Improve this Doc View SourceSetupGameObject()
RENDERING STEP 2. Take geometric rendering information computed in ComputeGeometry() and sets up proper game object(s) and components for this Data Impression. Transfers geometry into Unity format (e.g. a Mesh). No geometric computations should happen in this method, and it should generally be lightweight.
Declaration
public override void SetupGameObject()
Overrides
| Improve this Doc View SourceUpdateStyling()
RENDERING STEP 3. Update the "styling" of an impression by sending each styling parameter to the shader. Occasionally will need to set per-vertex items like transforms. This method should generally be lightweight.
Declaration
public override void UpdateStyling()
Overrides
| Improve this Doc View SourceUpdateVisibility()
RENDERING STEP 4. Update the visibility of an impression (hidden or shown)
Declaration
public override void UpdateVisibility()