Class SimpleLineDataImpression
A "Lines" data impression that uses hand-drawn line textures to depict line/flow data.
Namespace: IVLab.ABREngine
Assembly: IVLab.ABREngine.Runtime.dll
Syntax
[ABRPlateType("Ribbons")]
public class SimpleLineDataImpression : DataImpression, IHasKeyData, ICoordSpaceConverter, IHasDataset
Examples
An example of creating a single line data impression and setting its colormap, color variable, and line texture could be:
SimpleLineDataImpression gi = new SimpleLineDataImpression();
gi.keyData = lines;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.lineTexture = line;
ABREngine.Instance.RegisterDataImpression(gi);
Constructors
| Improve this Doc View SourceSimpleLineDataImpression()
A "Lines" data impression that uses hand-drawn line textures to depict line/flow data.
Declaration
protected SimpleLineDataImpression()
Examples
An example of creating a single line data impression and setting its colormap, color variable, and line texture could be:
SimpleLineDataImpression gi = new SimpleLineDataImpression();
gi.keyData = lines;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.lineTexture = line;
ABREngine.Instance.RegisterDataImpression(gi);
Fields
| Improve this Doc View SourceLayerName
Define the layer name for this Data Impression
Declaration
protected const string LayerName = "ABR_Line"
Field Value
Type | Description |
---|---|
string |
Remarks
Warning
New Data Impressions should define a const string "LayerName" which corresponds to a Layer in Unity's Layer manager.
averageCount
Number of "averaging" samples taken across the line for a smoothing effect. This example ranges from 0 to 50.
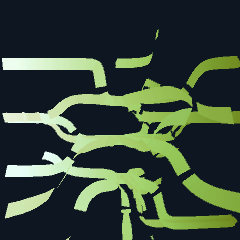
Declaration
[ABRInput("Ribbon Smooth", UpdateLevel.Geometry)]
public IntegerPrimitive averageCount
Field Value
Type | Description |
---|---|
IntegerPrimitive |
colorVariable
Scalar color variable applied to each point on the line of this data impression. This example switches between X-axis monotonically increasing and Y-axis monotonically increasing.
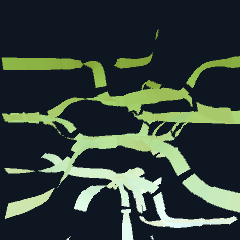
Declaration
[ABRInput("Color Variable", UpdateLevel.Style)]
public ScalarDataVariable colorVariable
Field Value
Type | Description |
---|---|
ScalarDataVariable |
colormap
Colormap applied to the colorVariable. This example switches between a linear white-to-green colormap and a linear black-to-white colormap.
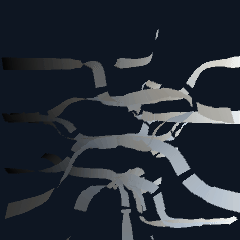
Declaration
[ABRInput("Colormap", UpdateLevel.Style)]
public IColormapVisAsset colormap
Field Value
Type | Description |
---|---|
IColormapVisAsset |
defaultCurveDirection
Change the default curvature axis (if there are no existing tangents on the curve, this axis will be used)
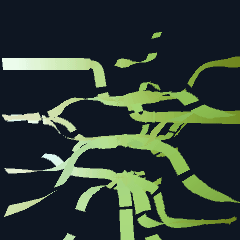
Declaration
public Vector3 defaultCurveDirection
Field Value
Type | Description |
---|---|
Vector3 |
Remarks
NOTE: This input mostly changes behaviour at the ends of ribbons, unless your ribbon is perfectly straight. (This setting exists because of perfectly straight ribbons which the existing ribbon has trouble with).
keyData
A "Lines" data impression that uses hand-drawn line textures to depict line/flow data.
Declaration
[ABRInput("Key Data", UpdateLevel.Geometry)]
public KeyData keyData
Field Value
Type | Description |
---|---|
KeyData |
Examples
An example of creating a single line data impression and setting its colormap, color variable, and line texture could be:
SimpleLineDataImpression gi = new SimpleLineDataImpression();
gi.keyData = lines;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.lineTexture = line;
ABREngine.Instance.RegisterDataImpression(gi);
|
Improve this Doc
View Source
lineTexture
Texture applied to the line. Light areas on the texture are
discarded, dark areas are kept. Can also be a LineTextureGradient
Declaration
[ABRInput("Texture", UpdateLevel.Style)]
public ILineTextureVisAsset lineTexture
Field Value
Type | Description |
---|---|
ILineTextureVisAsset |
lineTextureVariable
Scalar variable used to vary the line texture across its length.
Declaration
[ABRInput("Texture Variable", UpdateLevel.Style)]
public ScalarDataVariable lineTextureVariable
Field Value
Type | Description |
---|---|
ScalarDataVariable |
lineWidth
Width of the line, in Unity world units.
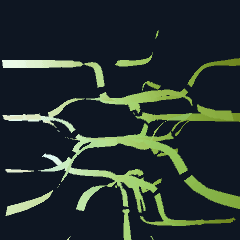
Declaration
[ABRInput("Ribbon Width", UpdateLevel.Geometry)]
public LengthPrimitive lineWidth
Field Value
Type | Description |
---|---|
LengthPrimitive |
nanColor
Override the color used for NaN values in this data impression. If not supplied, will use the defaultNanColor.
Declaration
[ABRInput("NaN Color", UpdateLevel.Style)]
public IColormapVisAsset nanColor
Field Value
Type | Description |
---|---|
IColormapVisAsset |
nanLineTexture
Override the line texture used for NaN values in this data impression. If not supplied, will use the defaultNanLine.
Declaration
[ABRInput("NaN Texture", UpdateLevel.Style)]
public ILineTextureVisAsset nanLineTexture
Field Value
Type | Description |
---|---|
ILineTextureVisAsset |
ribbonBrightness
Manually adjust the brightness of the ribbon regardless of lighting in the scene.
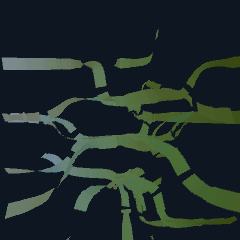
Declaration
[ABRInput("Ribbon Brightness", UpdateLevel.Style)]
public PercentPrimitive ribbonBrightness
Field Value
Type | Description |
---|---|
PercentPrimitive |
ribbonCurveAngle
Subtly adjust the lighting by varying the lighting normal of the ribbon
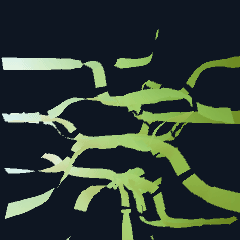
Declaration
[ABRInput("Ribbon Curve", UpdateLevel.Geometry)]
public AnglePrimitive ribbonCurveAngle
Field Value
Type | Description |
---|---|
AnglePrimitive |
ribbonRotationAngle
Rotate the ribbon along its central axis. This example goes from 0 degrees to 90 degrees.
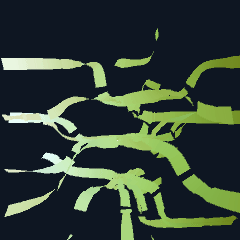
Declaration
[ABRInput("Ribbon Rotation", UpdateLevel.Geometry)]
public AnglePrimitive ribbonRotationAngle
Field Value
Type | Description |
---|---|
AnglePrimitive |
textureCutoff
"Cutoff" point for discarding portions of the line. The cutoff is between 0% (fully light) and 100% (fully dark). In practice, this is performing a threshold filter.
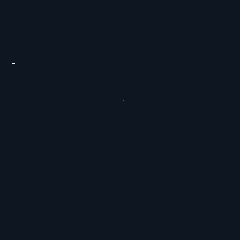
Declaration
[ABRInput("Texture Cutoff", UpdateLevel.Style)]
public PercentPrimitive textureCutoff
Field Value
Type | Description |
---|---|
PercentPrimitive |
Remarks
NOTE: This input will have no effect if there's no lineTexture applied. It has the most effect on textures that are not fully black/white.
Properties
| Improve this Doc View SourceMaterialNames
Name of the material to use to render this DataImpression
Declaration
protected override string[] MaterialNames { get; }
Property Value
Type | Description |
---|---|
string[] |
Overrides
Methods
| Improve this Doc View SourceComputeGeometry()
RENDERING STEP 1. Populate rendering information (Geometry) for the DataImpression. This is triggered by the DataImpressionGroup when an Geometry happens. This step is generally expensive.
Declaration
public override void ComputeGeometry()
Overrides
| Improve this Doc View SourceGetDataset()
By default, there's no dataset. DataImpressions should only have one dataset, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override Dataset GetDataset()
Returns
Type | Description |
---|---|
Dataset |
Overrides
| Improve this Doc View SourceGetKeyData()
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override KeyData GetKeyData()
Returns
Type | Description |
---|---|
KeyData |
Overrides
| Improve this Doc View SourceGetKeyDataTopology()
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override DataTopology GetKeyDataTopology()
Returns
Type | Description |
---|---|
DataTopology |
Overrides
| Improve this Doc View SourceSetKeyData(KeyData)
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override void SetKeyData(KeyData kd)
Parameters
Type | Name | Description |
---|---|---|
KeyData | kd |
Overrides
| Improve this Doc View SourceSetupGameObject()
RENDERING STEP 2. Take geometric rendering information computed in ComputeGeometry() and sets up proper game object(s) and components for this Data Impression. Transfers geometry into Unity format (e.g. a Mesh). No geometric computations should happen in this method, and it should generally be lightweight.
Declaration
public override void SetupGameObject()
Overrides
| Improve this Doc View SourceUpdateStyling()
RENDERING STEP 3. Update the "styling" of an impression by sending each styling parameter to the shader. Occasionally will need to set per-vertex items like transforms. This method should generally be lightweight.
Declaration
public override void UpdateStyling()
Overrides
| Improve this Doc View SourceUpdateVisibility()
RENDERING STEP 4. Update the visibility of an impression (hidden or shown)
Declaration
public override void UpdateVisibility()