Class SimpleVolumeDataImpression
A "Volumes" data impression that uses a user-defined transfer (opacity) map and a colormap to show volumetric data.
Inheritance
Namespace: IVLab.ABREngine
Assembly: IVLab.ABREngine.Runtime.dll
Syntax
[ABRPlateType("Volumes")]
public class SimpleVolumeDataImpression : DataImpression, IHasDataset, IHasKeyData, ICoordSpaceConverter
Examples
An example of creating a single volume data impression and setting its colormap and opacity map could be:
SimpleVolumeDataImpression gi = new SimpleVolumeDataImpression();
gi.keyData = volume;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.opacityMap = PrimitiveGradient.Default();
ABREngine.Instance.RegisterDataImpression(gi);
Constructors
| Improve this Doc View SourceSimpleVolumeDataImpression()
A "Volumes" data impression that uses a user-defined transfer (opacity) map and a colormap to show volumetric data.
Declaration
protected SimpleVolumeDataImpression()
Examples
An example of creating a single volume data impression and setting its colormap and opacity map could be:
SimpleVolumeDataImpression gi = new SimpleVolumeDataImpression();
gi.keyData = volume;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.opacityMap = PrimitiveGradient.Default();
ABREngine.Instance.RegisterDataImpression(gi);
Fields
| Improve this Doc View SourceLayerName
Define the layer name for this Data Impression
Declaration
protected const string LayerName = "ABR_Volume"
Field Value
Type | Description |
---|---|
string |
Remarks
Warning
New Data Impressions should define a const string "LayerName" which corresponds to a Layer in Unity's Layer manager.
colorVariable
Scalar color variable applied to each voxel of this data impression
- affects both the colormap and the opacitymap.
Declaration
[ABRInput("Color Variable", UpdateLevel.Geometry)]
public ScalarDataVariable colorVariable
Field Value
Type | Description |
---|---|
ScalarDataVariable |
colormap
Colormap applied to the colorVariable. This example switches between a linear white-to-green colormap and a linear black-to-white colormap.
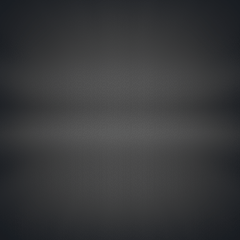
Declaration
[ABRInput("Colormap", UpdateLevel.Style)]
public IColormapVisAsset colormap
Field Value
Type | Description |
---|---|
IColormapVisAsset |
keyData
A "Volumes" data impression that uses a user-defined transfer (opacity) map and a colormap to show volumetric data.
Declaration
[ABRInput("Key Data", UpdateLevel.Geometry)]
public KeyData keyData
Field Value
Type | Description |
---|---|
KeyData |
Examples
An example of creating a single volume data impression and setting its colormap and opacity map could be:
SimpleVolumeDataImpression gi = new SimpleVolumeDataImpression();
gi.keyData = volume;
gi.colorVariable = yAxis;
gi.colormap = ABREngine.Instance.VisAssets.GetDefault<ColormapVisAsset>() as ColormapVisAsset;
gi.opacityMap = PrimitiveGradient.Default();
ABREngine.Instance.RegisterDataImpression(gi);
|
Improve this Doc
View Source
nanColor
Override the color used for NaN values in this data impression. If not supplied, will use the defaultNanColor.
Declaration
[ABRInput("NaN Color", UpdateLevel.Style)]
public IColormapVisAsset nanColor
Field Value
Type | Description |
---|---|
IColormapVisAsset |
nanOpacity
Override the color used for NaN values in this data impression. If not supplied, will be 0% opacity.
Declaration
public PercentPrimitive nanOpacity
Field Value
Type | Description |
---|---|
PercentPrimitive |
opacitymap
The real power of volume rendering is in the opacity map, or transfer function.
For example, with a "spike" transfer function changing over time like this, we can achieve a sort of contour or isosurface scanning through the volume.
Declaration
[ABRInput("Opacitymap", UpdateLevel.Style)]
public PrimitiveGradient opacitymap
Field Value
Type | Description |
---|---|
PrimitiveGradient |
volumeBrightness
Brightness multiplier for the entire volume, irrespective of lighting.
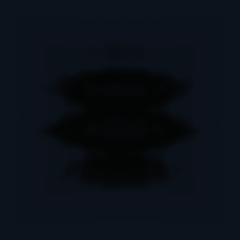
Declaration
[ABRInput("Volume Brightness", UpdateLevel.Style)]
public PercentPrimitive volumeBrightness
Field Value
Type | Description |
---|---|
PercentPrimitive |
volumeLighting
Should the current scene's lighting affect the volume or not?
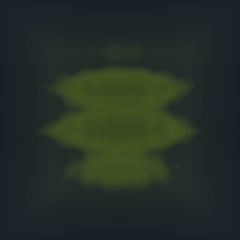
Declaration
[ABRInput("Volume Lighting", UpdateLevel.Style)]
public BooleanPrimitive volumeLighting
Field Value
Type | Description |
---|---|
BooleanPrimitive |
Remarks
Lighting is often useful for understanding 3D structures and creating atmospheric effects, but may not be useful for nitty-gritty data interpretation.
volumeOpacityMultiplier
Opacity multiplier for the entire volume; gets multiplied on top of the opacitymap.
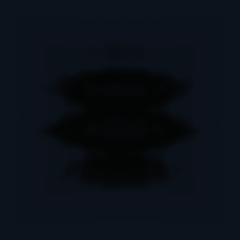
Declaration
[ABRInput("Volume Opacity Multiplier", UpdateLevel.Style)]
public PercentPrimitive volumeOpacityMultiplier
Field Value
Type | Description |
---|---|
PercentPrimitive |
Properties
| Improve this Doc View SourceMaterialNames
Name of the material to use to render this DataImpression
Declaration
protected override string[] MaterialNames { get; }
Property Value
Type | Description |
---|---|
string[] |
Overrides
Methods
| Improve this Doc View SourceComputeGeometry()
RENDERING STEP 1. Populate rendering information (Geometry) for the DataImpression. This is triggered by the DataImpressionGroup when an Geometry happens. This step is generally expensive.
Declaration
public override void ComputeGeometry()
Overrides
| Improve this Doc View SourceGetDataset()
By default, there's no dataset. DataImpressions should only have one dataset, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override Dataset GetDataset()
Returns
Type | Description |
---|---|
Dataset |
Overrides
| Improve this Doc View SourceGetKeyData()
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override KeyData GetKeyData()
Returns
Type | Description |
---|---|
KeyData |
Overrides
| Improve this Doc View SourceGetKeyDataTopology()
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override DataTopology GetKeyDataTopology()
Returns
Type | Description |
---|---|
DataTopology |
Overrides
| Improve this Doc View SourceSetKeyData(KeyData)
By default, there's no data. DataImpressions should only have one KeyData, and it's up to them individually to enforce that they correctly implement this.
Declaration
public override void SetKeyData(KeyData kd)
Parameters
Type | Name | Description |
---|---|---|
KeyData | kd |
Overrides
| Improve this Doc View SourceSetupGameObject()
RENDERING STEP 2. Take geometric rendering information computed in ComputeGeometry() and sets up proper game object(s) and components for this Data Impression. Transfers geometry into Unity format (e.g. a Mesh). No geometric computations should happen in this method, and it should generally be lightweight.
Declaration
public override void SetupGameObject()
Overrides
| Improve this Doc View SourceUpdateStyling()
RENDERING STEP 3. Update the "styling" of an impression by sending each styling parameter to the shader. Occasionally will need to set per-vertex items like transforms. This method should generally be lightweight.
Declaration
public override void UpdateStyling()
Overrides
| Improve this Doc View SourceUpdateVisibility()
RENDERING STEP 4. Update the visibility of an impression (hidden or shown)
Declaration
public override void UpdateVisibility()